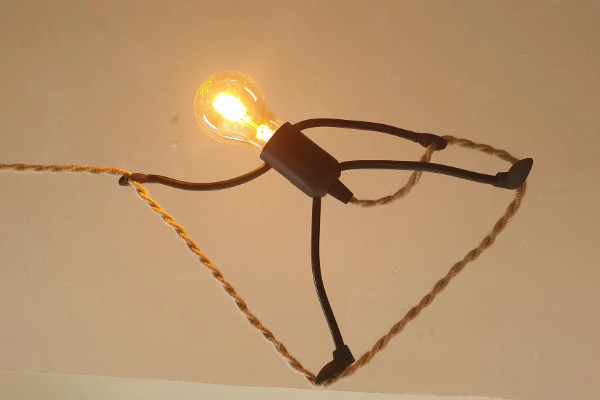
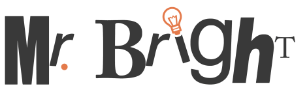
Met Mr. Bright komt licht tot leven.
En dat wordt niet alleen zichtbaar in de producten zelf!
Want:
- De montage van Mr.Bright gebeurt in een sociale werkplaats.
- En Mr.Bright staat voor een charme offensief tegen ‘lichtarmoede’ wereldwijd.
Hallo daar! Wat leuk dat je er bent! Ik ben Mr.Bright. Een simpel maar slim ontwerp. Met de kracht van eenvoud en toch ongelofelijk veelzijdig. Wie mij ziet schiet spontaan in de lach. En voelt zich meteen geinspireerd om creatief met mij aan de slag te gaan.
En het doet mij veel plezier als ik jouw huis, jouw leven daarmee mag komen verlichten. Want door in jouw leven te zijn geef ik meteen ook betekenis aan dat van vele anderen!
Ik word namelijk met de hand gemaakt. Bij stichting ’t Lichtpunt in Gouda. Door mensen die om verschillende redenen (nog) niet mee kunnen doen in een reguliere baan. Ik verbind deze mensen met elkaar, en biedt hen een dagelijkse structuur met een leuke activiteit om aan deel te nemen Ik ben daarmee dus een belangrijke bijdrage aan hun leven.
Daarnaast sta ik garant voor een charme-offensief tegen lichtarmoede in de wereld. Een deel van mijn opbrengst doneer ik aan het solar project van Stichting Een Wereld Idee. Want licht brengt leven!
Wereldwijd zijn er vele mensen die geen toegang hebben tot het reguliere elektriciteitsnet. Of afhankelijk zijn van een gebrekkige en onvoorspelbare stroomvoorziening. Als alternatief gebruiken ze vaak petroleum- of kerosinelampen. Deze lampen zijn duur in gebruik, geven maar weinig licht, en zijn bovendien een bedreiging voor de gezondheid. Ze zijn brandgevaarlijk, en de dampen zijn bijzonder slecht voor mens, dier en milieu. Het gebruik van zonnepanelen is een prachtige oplossing voor al deze problemen. En brengt zelfs nog zoveel meer dan alleen licht!
Als je daar meer over wilt weten, neem dan eens een kijkje op www.1we.org/solar-project voor uitgebreider informatie. Stichting Een Wereld Idee heeft trouwens, naast het solar project, ook nog vele andere interessante projecten lopen.
Wat ik hier maar mee wil zeggen; door simpelweg mezelf te zijn, met de kracht van eenvoud die mij zo kenmerkt, heb ik al veel heb weten te bereiken! En ik nodig je van harte uit om met me mee te wandelen op mijn ‘pad naar verlichting’. Want samen kunnen we een andere wereld creeen, jij en ik. Ga je mee op avontuur?
Met Mr. Bright draag je actief bij aan een betere wereld!
Gegevens Mr. Bright
Wie doet wat?
- Verzenden producten
- Bieden wettelijke garantie
- Online bestellen
- Veilig betalen
Filter
Collectie Mr. Bright